Barcode scanning has become an integral part of modern businesses today, helping in inventory management, sales tracking, and production processes. With the latest feature of AVFoundation, barcode scanning has become simpler and more efficient in iOS8 environment. In this article, we will talk about how to implement barcode scanning using the AVFoundation framework and Swift programming language.
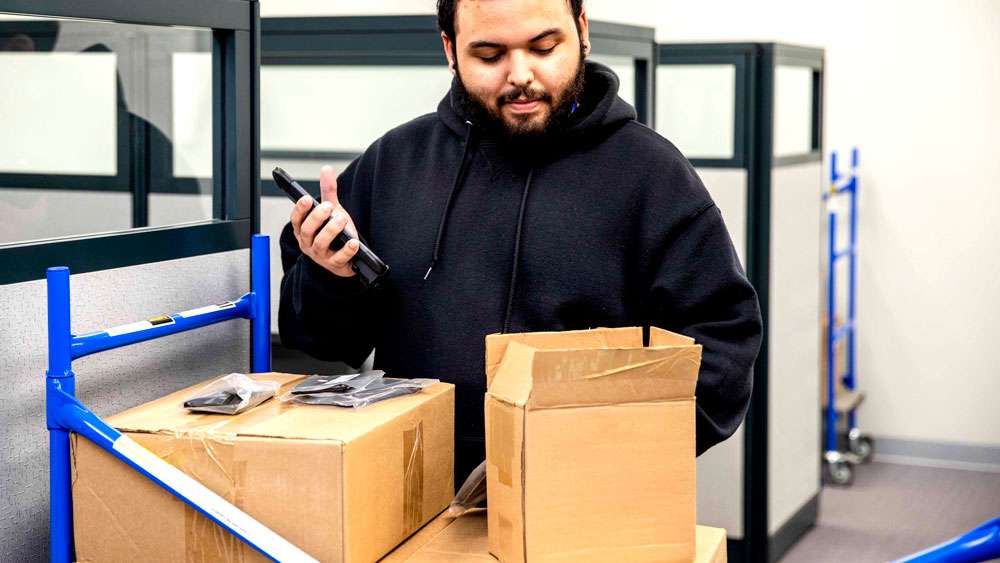
Before we dive deep into the technicalities of implementing barcode scanning, let's define what barcode scanning is and what are its benefits.
What is Barcode Scanning?
Barcode scanning simply refers to the process of reading machine-readable code in the form of a series of lines or dots. This code, known as a barcode, holds information about the product, such as its name, price, dimensions, and weight, and allows businesses to manage their inventory and customer data more effectively.
Benefits of Barcode Scanning
Barcode scanning has multiple benefits associated with it, some of which are:
1. Speed: Barcode scanning is a faster mode of collecting data as compared to manual data entry. It can save time and increase the overall efficiency of business processes.
2. Accuracy: Barcode scanning reduces human errors, which can occur during manual data entry.
3. Cost-effective: Barcode scanning requires minimal investment and can help businesses save money by reducing errors and increasing efficiency.
Now that we understand what barcode scanning is and why it's essential, let us go through the steps involved in implementing barcode scanning using the AVFoundation framework.
Step 1: Import AVFoundation Framework
To begin with, we need to import the AVFoundation framework to scan barcodes. The AVFoundation framework provides high-level interface and abstracts all the complex low-level mechanics that make scan possible. To start, we need to add the following import statement at the beginning of our ViewController.
```
import UIKit
import AVFoundation
```
Step 2: Set up AVCaptureSession
The next step is to initialize a capturing session for video input using AVCaptureSession. The AVCaptureSession allows capturing and processing video data for a given device input and outputs video frames to a given output. We need to use this session to capture video data and extract the barcode information.
```
let session = AVCaptureSession()
session.sessionPreset = AVCaptureSession.Preset.photo
```
Step 3: Add Video Input and Output
Once we have the capturing session, we need to add the video input to this session. We will use AVCaptureDeviceInput to initialize our video input. We also need to set the output where we will get the barcode information. For this, we will use AVCaptureMetadataOutput.
```
guard let captureDevice = AVCaptureDevice.default(for: .video) else { return }
let videoInput: AVCaptureDeviceInput
do {
videoInput = try AVCaptureDeviceInput(device: captureDevice)
} catch {
return
}
if (session.canAddInput(videoInput)) {
session.addInput(videoInput)
}
let metaDataOutput = AVCaptureMetadataOutput()
if (session.canAddOutput(metaDataOutput)) {
session.addOutput(metaDataOutput)
metaDataOutput.setMetadataObjectsDelegate(self, queue: DispatchQueue.main)
metaDataOutput.metadataObjectTypes = [.qr, .ean8, .ean13, .pdf417]
}
```
Step 4: Start Capturing Session
Now that we have set up our capturing session, we can start capturing the video by calling the startRunning method on our AVCaptureSession object.
```
session.startRunning()
```
Step 5: Exract Barcode Data
The final step involves extracting the barcode data that has been captured by the camera. We can do this via the AVCaptureMetadataOutputObjectsDelegate delegate method, which captures the metadata objects that have been recognized by the video output.
```
extension ViewController: AVCaptureMetadataOutputObjectsDelegate {
func metadataOutput(_ output: AVCaptureMetadataOutput, didOutput metadataObjects: [AVMetadataObject], from connection: AVCaptureConnection) {
guard let metadataObject = metadataObjects.first else {
return
}
guard let readableObject = metadataObject as? AVMetadataMachineReadableCodeObject else {
return
}
let barcode = readableObject.stringValue ?? ""
print("Barcode Data: \(barcode)")
}
}
```
This method captures the first metadata object and then checks if it is a machine-readable code object. If yes, we get the barcode value from it and print it to the console.
Conclusion
In this article, we have learned about the basic steps involved in implementing barcode scanning in iOS8 using Swift programming language and AVFoundation framework. We also discussed the benefits of barcode scanning and how it can help businesses become more efficient and cost-effective. With this knowledge, you can start exploring more complex use cases and develop your own barcode scanner application using these frameworks. The possibilities are endless; all you need is a little creativity and effort.